Cloned Web Ignore List
We have added the ability to add your own regex or website to be ignored when alerting for Cloned web Canarytokens.
This is useful if you see that your Cloned Web token is generating alerts that you deem "safe".
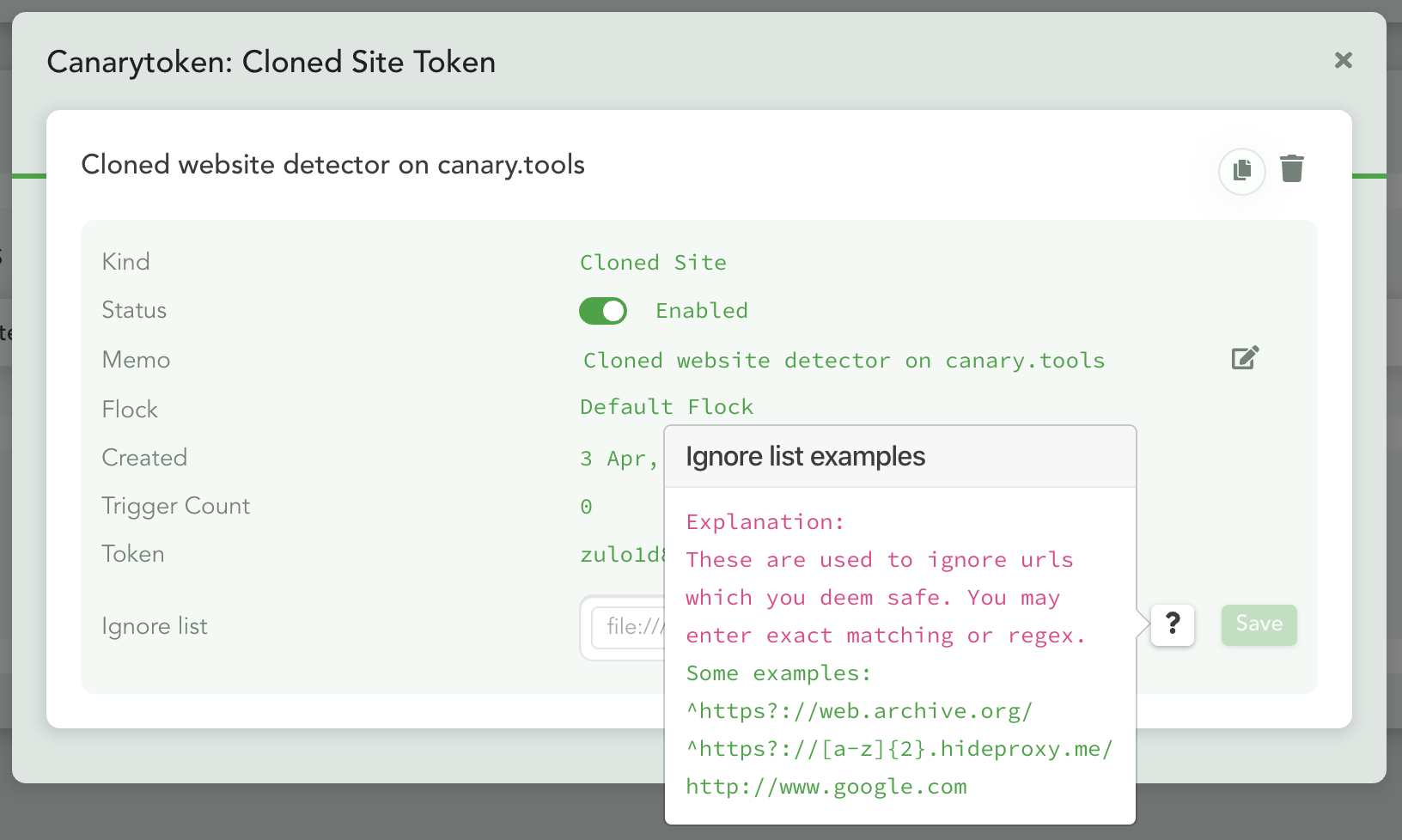
Disable Ignore List
POST /api/v1/canarytokens/clonedsite/ignorelist/disable
Disable the ignore list for a specific Cloned Web token.
Required Parameters
auth_token string
A valid auth token
flock_id string
A valid flock_id
canarytoken string
A valid Canarytoken
Response
A JSON structure with result indicator.
Example
curl https://EXAMPLE.canary.tools/api/v1/canarytokens/clonedsite/ignorelist/disable \
-d auth_token=EXAMPLE_AUTH_TOKEN \
-d flock_id=flock:default \
-d canarytoken=EXAMPLE_CANARYTOKEN
1
2
3
4
import requests
url = 'https://EXAMPLE.canary.tools/api/v1/canarytokens/clonedsite/ignorelist/disable'
payload = {
'auth_token': 'EXAMPLE_AUTH_TOKEN',
'flock_id': 'flock:default',
'canarytoken': 'EXAMPLE_CANARYTOKEN'
}
r = requests.post(url, data=payload)
print(r.json())
1
2
3
4
5
6
7
8
9
10
11
12
13
{
"result": "success"
}
1
2
3
Enable Ignore List
POST /api/v1/canarytokens/clonedsite/ignorelist/enable
Enable the ignore list for a specific Cloned Web token.
Required Parameters
auth_token string
A valid auth token
flock_id string
A valid flock_id
canarytoken string
A valid Canarytoken
Response
A JSON structure with result indicator.
Example
curl https://EXAMPLE.canary.tools/api/v1/canarytokens/clonedsite/ignorelist/enable \
-d auth_token=EXAMPLE_AUTH_TOKEN \
-d flock_id=flock:default \
-d canarytoken=EXAMPLE_CANARYTOKEN
1
2
3
4
import requests
url = 'https://EXAMPLE.canary.tools/api/v1/canarytokens/clonedsite/ignorelist/enable'
payload = {
'auth_token': 'EXAMPLE_AUTH_TOKEN',
'flock_id': 'flock:default',
'canarytoken': 'EXAMPLE_CANARYTOKEN'
}
r = requests.post(url, data=payload)
print(r.json())
1
2
3
4
5
6
7
8
9
10
11
12
13
{
"result": "success"
}
1
2
3
Fetch Ignore List
GET /api/v1/canarytokens/clonedsite/ignorelist/fetch
Enable the ignore list for a specific Cloned Web token.
Required Parameters
auth_token string
A valid auth token
flock_id string
A valid flock_id
canarytoken string
A valid Canarytoken
Response
A JSON structure containing the ignore list info for the specified Cloned Web token.
Example
curl https://EXAMPLE.canary.tools/api/v1/canarytokens/clonedsite/ignorelist/fetch \
-d auth_token=EXAMPLE_AUTH_TOKEN \
-d flock_id=flock:default \
-d canarytoken=EXAMPLE_CANARYTOKEN \
-G
1
2
3
4
5
import requests
url = 'https://EXAMPLE.canary.tools/api/v1/canarytokens/clonedsite/ignorelist/fetch'
payload = {
'auth_token': 'EXAMPLE_AUTH_TOKEN',
'flock_id': 'flock:default',
'canarytoken': 'EXAMPLE_CANARYTOKEN'
}
r = requests.get(url, params=payload)
print(r.json())
1
2
3
4
5
6
7
8
9
10
11
12
13
{
"ignore_lists": {
"enabled": "true",
"ignores": "^https?://web.archive.org,^https?://[a-z]{2}.hideproxy.me/"
},
"result": "success"
}
1
2
3
4
5
6
7
Save Ignore List
POST /api/v1/canarytokens/clonedsite/ignorelist/save
Save a new ignore list for a specific Cloned Web token.
Required Parameters
auth_token string
A valid auth token
flock_id string
A valid flock_id
canarytoken string
A valid Canarytoken
Optional Parameters
ignores string
A comma separated list of regex ignores
WARNING
Sending through a save without ignores
, will assume you want to blank previously set values.
Response
A JSON structure with result indicator.
Example
curl https://EXAMPLE.canary.tools/api/v1/canarytokens/clonedsite/ignorelist/save \
-d auth_token=EXAMPLE_AUTH_TOKEN \
-d flock_id=flock:default \
-d canarytoken=EXAMPLE_CANARYTOKEN \
-d ignores='^https?://web.archive.org','^https?://[a-z]{2}.hideproxy.me/'
1
2
3
4
5
import requests
url = 'https://EXAMPLE.canary.tools/api/v1/canarytokens/clonedsite/ignorelist/save'
payload = {
'auth_token': 'EXAMPLE_AUTH_TOKEN',
'flock_id': 'flock:default',
'canarytoken': 'EXAMPLE_CANARYTOKEN',
'ignores':'^https?://web.archive.org,^https?://[a-z]{2}.hideproxy.me/'
}
r = requests.post(url, data=payload)
print(r.json())
1
2
3
4
5
6
7
8
9
10
11
12
13
14
{
"result": "success"
}
1
2
3